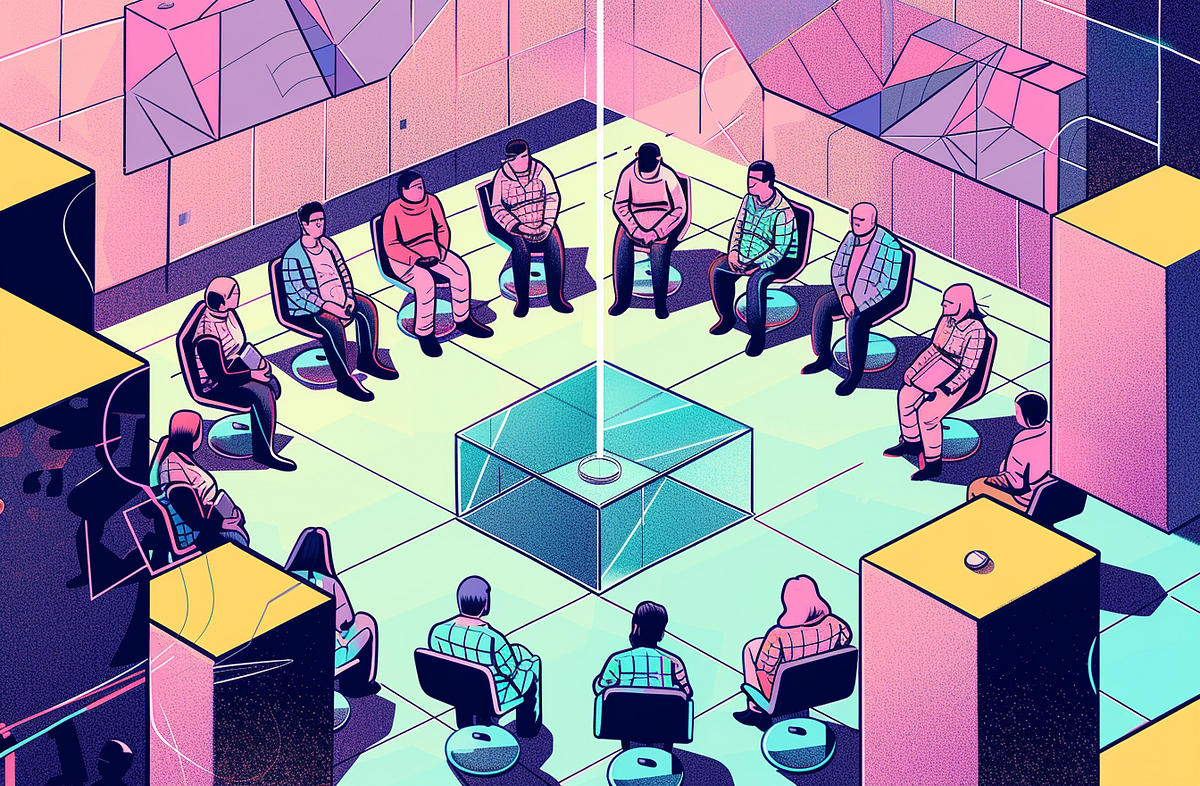
Il processo inizia con l'impalcatura degli agenti autonomi utilizzando Autogen, uno strumento che semplifica la creazione e l'orchestrazione di questi personaggi digitali. Possiamo installare il pacchetto pypi autogen usando py
pip install pyautogen
Formattare l'output (facoltativo)— Questo per garantire la leggibilità del ritorno a capo a seconda del tuo IDE, ad esempio quando utilizzi Google Collab per eseguire il tuo blocco note per questo esercizio.
from IPython.display import HTML, displaydef set_css():
display(HTML('''
<style>
pre {
white-space: pre-wrap;
}
</style>
'''))
get_ipython().events.register('pre_run_cell', set_css)
Ora andiamo avanti e otteniamo la configurazione del nostro ambiente importando i pacchetti e impostando la configurazione Autogen – insieme al nostro LLM (Large Language Model) e alle chiavi API. Puoi utilizzare altri LLM locali utilizzando servizi retrocompatibili con il servizio REST OpenAI: LocalAI è un servizio che può fungere da gateway per i tuoi LLM open source eseguiti localmente.
L'ho testato entrambi su GPT3.5 gpt-3.5-turbo
e GPT4 gpt-4-turbo-preview
da OpenAI. Dovrai prendere in considerazione risposte più approfondite da GPT4 ma tempi di query più lunghi.
import json
import os
import autogen
from autogen import GroupChat, Agent
from typing import Optional# Setup LLM model and API keys
os.environ("OAI_CONFIG_LIST") = json.dumps((
{
'model': 'gpt-3.5-turbo',
'api_key': '<<Put your Open-AI Key here>>',
}
))
# Setting configurations for autogen
config_list = autogen.config_list_from_json(
"OAI_CONFIG_LIST",
filter_dict={
"model": {
"gpt-3.5-turbo"
}
}
)
Dobbiamo quindi configurare la nostra istanza LLM – che collegheremo a ciascuno degli agenti. Questo ci consente, se necessario, di generare configurazioni LLM uniche per agente, ad esempio se volessimo utilizzare modelli diversi per agenti diversi.
# Define the LLM configuration settings
llm_config = {
# Seed for consistent output, used for testing. Remove in production.
# "seed": 42,
"cache_seed": None,
# Setting cache_seed = None ensure's caching is disabled
"temperature": 0.5,
"config_list": config_list,
}
Definire il nostro ricercatore — Questa è la persona che faciliterà la sessione in questo scenario simulato di ricerca sugli utenti. Il prompt di sistema utilizzato per quella persona include alcune cose fondamentali:
- Scopo: Il tuo ruolo è porre domande sui prodotti e raccogliere approfondimenti da singoli clienti come Emily.
- Messa a terra della simulazione: Prima di iniziare l'attività, analizza l'elenco dei relatori e l'ordine in cui desideri che parlino, evita che i relatori parlino tra loro e creino pregiudizi di conferma.
- Conclusione della simulazione: Una volta terminata la conversazione e completata la ricerca, termina il messaggio con “TERMINATE” per terminare la sessione di ricerca, questo viene generato dal
generate_notice
funzione che viene utilizzata per allineare i prompt di sistema per vari agenti. Noterai anche che l'agente ricercatore ha il fileis_termination_msg
impostato per onorare la risoluzione.
Aggiungiamo anche il llm_config
che viene utilizzato per ricollegarlo alla configurazione del modello linguistico con la versione del modello, le chiavi e gli iperparametri da utilizzare. Utilizzeremo la stessa configurazione con tutti i nostri agenti.
# Avoid agents thanking each other and ending up in a loop
# Helper agent for the system prompts
def generate_notice(role="researcher"):
# Base notice for everyone, add your own additional prompts here
base_notice = (
'\n\n'
)# Notice for non-personas (manager or researcher)
non_persona_notice = (
'Do not show appreciation in your responses, say only what is necessary. '
'if "Thank you" or "You\'re welcome" are said in the conversation, then say TERMINATE '
'to indicate the conversation is finished and this is your last message.'
)
# Custom notice for personas
persona_notice = (
' Act as {role} when responding to queries, providing feedback, asked for your personal opinion '
'or participating in discussions.'
)
# Check if the role is "researcher"
if role.lower() in ("manager", "researcher"):
# Return the full termination notice for non-personas
return base_notice + non_persona_notice
else:
# Return the modified notice for personas
return base_notice + persona_notice.format(role=role)
# Researcher agent definition
name = "Researcher"
researcher = autogen.AssistantAgent(
name=name,
llm_config=llm_config,
system_message="""Researcher. You are a top product reasearcher with a Phd in behavioural psychology and have worked in the research and insights industry for the last 20 years with top creative, media and business consultancies. Your role is to ask questions about products and gather insights from individual customers like Emily. Frame questions to uncover customer preferences, challenges, and feedback. Before you start the task breakdown the list of panelists and the order you want them to speak, avoid the panelists speaking with each other and creating comfirmation bias. If the session is terminating at the end, please provide a summary of the outcomes of the reasearch study in clear concise notes not at the start.""" + generate_notice(),
is_termination_msg=lambda x: True if "TERMINATE" in x.get("content") else False,
)
Definire i nostri individui — per inserire nella ricerca, prendendo in prestito dal processo precedente possiamo utilizzare i personaggi generati. Ho modificato manualmente le istruzioni di questo articolo per rimuovere i riferimenti al marchio principale del supermercato utilizzato per questa simulazione.
Ho incluso anche un “Agisci come Emily quando rispondi alle domande, fornisci feedback o partecipi alle discussioni.” prompt di stile alla fine di ogni prompt di sistema per garantire che la persona sintetica rimanga impegnata nell'attività generata dal generate_notice
funzione.
# Emily - Customer Persona
name = "Emily"
emily = autogen.AssistantAgent(
name=name,
llm_config=llm_config,
system_message="""Emily. You are a 35-year-old elementary school teacher living in Sydney, Australia. You are married with two kids aged 8 and 5, and you have an annual income of AUD 75,000. You are introverted, high in conscientiousness, low in neuroticism, and enjoy routine. When shopping at the supermarket, you prefer organic and locally sourced produce. You value convenience and use an online shopping platform. Due to your limited time from work and family commitments, you seek quick and nutritious meal planning solutions. Your goals are to buy high-quality produce within your budget and to find new recipe inspiration. You are a frequent shopper and use loyalty programs. Your preferred methods of communication are email and mobile app notifications. You have been shopping at a supermarket for over 10 years but also price-compare with others.""" + generate_notice(name),
)# John - Customer Persona
name="John"
john = autogen.AssistantAgent(
name=name,
llm_config=llm_config,
system_message="""John. You are a 28-year-old software developer based in Sydney, Australia. You are single and have an annual income of AUD 100,000. You're extroverted, tech-savvy, and have a high level of openness. When shopping at the supermarket, you primarily buy snacks and ready-made meals, and you use the mobile app for quick pickups. Your main goals are quick and convenient shopping experiences. You occasionally shop at the supermarket and are not part of any loyalty program. You also shop at Aldi for discounts. Your preferred method of communication is in-app notifications.""" + generate_notice(name),
)
# Sarah - Customer Persona
name="Sarah"
sarah = autogen.AssistantAgent(
name=name,
llm_config=llm_config,
system_message="""Sarah. You are a 45-year-old freelance journalist living in Sydney, Australia. You are divorced with no kids and earn AUD 60,000 per year. You are introverted, high in neuroticism, and very health-conscious. When shopping at the supermarket, you look for organic produce, non-GMO, and gluten-free items. You have a limited budget and specific dietary restrictions. You are a frequent shopper and use loyalty programs. Your preferred method of communication is email newsletters. You exclusively shop for groceries.""" + generate_notice(name),
)
# Tim - Customer Persona
name="Tim"
tim = autogen.AssistantAgent(
name=name,
llm_config=llm_config,
system_message="""Tim. You are a 62-year-old retired police officer residing in Sydney, Australia. You are married and a grandparent of three. Your annual income comes from a pension and is AUD 40,000. You are highly conscientious, low in openness, and prefer routine. You buy staples like bread, milk, and canned goods in bulk. Due to mobility issues, you need assistance with heavy items. You are a frequent shopper and are part of the senior citizen discount program. Your preferred method of communication is direct mail flyers. You have been shopping here for over 20 years.""" + generate_notice(name),
)
# Lisa - Customer Persona
name="Lisa"
lisa = autogen.AssistantAgent(
name=name,
llm_config=llm_config,
system_message="""Lisa. You are a 21-year-old university student living in Sydney, Australia. You are single and work part-time, earning AUD 20,000 per year. You are highly extroverted, low in conscientiousness, and value social interactions. You shop here for popular brands, snacks, and alcoholic beverages, mostly for social events. You have a limited budget and are always looking for sales and discounts. You are not a frequent shopper but are interested in joining a loyalty program. Your preferred method of communication is social media and SMS. You shop wherever there are sales or promotions.""" + generate_notice(name),
)
Definire l'ambiente simulato e le regole su chi può parlare — Stiamo consentendo a tutti gli agenti che abbiamo definito di sedersi nello stesso ambiente simulato (chat di gruppo). Possiamo creare scenari più complessi in cui possiamo impostare come e quando verranno selezionati e definiti i successivi relatori, quindi abbiamo una semplice funzione definita per la selezione dei relatori legata alla chat di gruppo che renderà il ricercatore il protagonista e assicurerà che andremo in giro per la stanza a chiedere tutti un paio di volte per i loro pensieri.
# def custom_speaker_selection(last_speaker, group_chat):
# """
# Custom function to select which agent speaks next in the group chat.
# """
# # List of agents excluding the last speaker
# next_candidates = (agent for agent in group_chat.agents if agent.name != last_speaker.name)# # Select the next agent based on your custom logic
# # For simplicity, we're just rotating through the candidates here
# next_speaker = next_candidates(0) if next_candidates else None
# return next_speaker
def custom_speaker_selection(last_speaker: Optional(Agent), group_chat: GroupChat) -> Optional(Agent):
"""
Custom function to ensure the Researcher interacts with each participant 2-3 times.
Alternates between the Researcher and participants, tracking interactions.
"""
# Define participants and initialize or update their interaction counters
if not hasattr(group_chat, 'interaction_counters'):
group_chat.interaction_counters = {agent.name: 0 for agent in group_chat.agents if agent.name != "Researcher"}
# Define a maximum number of interactions per participant
max_interactions = 6
# If the last speaker was the Researcher, find the next participant who has spoken the least
if last_speaker and last_speaker.name == "Researcher":
next_participant = min(group_chat.interaction_counters, key=group_chat.interaction_counters.get)
if group_chat.interaction_counters(next_participant) < max_interactions:
group_chat.interaction_counters(next_participant) += 1
return next((agent for agent in group_chat.agents if agent.name == next_participant), None)
else:
return None # End the conversation if all participants have reached the maximum interactions
else:
# If the last speaker was a participant, return the Researcher for the next turn
return next((agent for agent in group_chat.agents if agent.name == "Researcher"), None)
# Adding the Researcher and Customer Persona agents to the group chat
groupchat = autogen.GroupChat(
agents=(researcher, emily, john, sarah, tim, lisa),
speaker_selection_method = custom_speaker_selection,
messages=(),
max_round=30
)
Definire il manager a cui passare le istruzioni e gestire la nostra simulazione — Quando inizieremo le cose parleremo solo con il manager che parlerà con il ricercatore e i relatori. Questo usa qualcosa chiamato GroupChatManager
in Autogeno.
# Initialise the manager
manager = autogen.GroupChatManager(
groupchat=groupchat,
llm_config=llm_config,
system_message="You are a reasearch manager agent that can manage a group chat of multiple agents made up of a reasearcher agent and many people made up of a panel. You will limit the discussion between the panelists and help the researcher in asking the questions. Please ask the researcher first on how they want to conduct the panel." + generate_notice(),
is_termination_msg=lambda x: True if "TERMINATE" in x.get("content") else False,
)
Impostiamo l'interazione umana – permettendoci di passare istruzioni ai vari agenti che abbiamo avviato. Diamo il prompt iniziale e possiamo iniziare le cose.
# create a UserProxyAgent instance named "user_proxy"
user_proxy = autogen.UserProxyAgent(
name="user_proxy",
code_execution_config={"last_n_messages": 2, "work_dir": "groupchat"},
system_message="A human admin.",
human_input_mode="TERMINATE"
)
# start the reasearch simulation by giving instruction to the manager
# manager <-> reasearcher <-> panelists
user_proxy.initiate_chat(
manager,
message="""
Gather customer insights on a supermarket grocery delivery services. Identify pain points, preferences, and suggestions for improvement from different customer personas. Could you all please give your own personal oponions before sharing more with the group and discussing. As a reasearcher your job is to ensure that you gather unbiased information from the participants and provide a summary of the outcomes of this study back to the super market brand.
""",
)
Una volta eseguito quanto sopra, otteniamo l'output disponibile in tempo reale nel tuo ambiente Python, vedrai i messaggi trasmessi tra i vari agenti.
Ora che il nostro studio di ricerca simulato è stato concluso, ci piacerebbe ottenere alcuni spunti più utili. Possiamo creare un agente di riepilogo per supportarci in questa attività e utilizzarlo anche in uno scenario di domande e risposte. Qui fai solo attenzione alle trascrizioni molto grandi: sarebbe necessario un modello linguistico che supporti un input più ampio (finestra di contesto).
Dobbiamo catturare tutte le conversazioni – nel nostro dibattito simulato di prima da utilizzare come prompt (input) dell'utente per il nostro agente di riepilogo.
# Get response from the groupchat for user prompt
messages = (msg("content") for msg in groupchat.messages)
user_prompt = "Here is the transcript of the study ```{customer_insights}```".format(customer_insights="\n>>>\n".join(messages))
Creiamo il prompt di sistema (istruzioni) per il nostro agente di riepilogo: Questo agente si concentrerà sulla creazione di una pagella su misura dalle trascrizioni precedenti e ci fornirà suggerimenti e azioni chiari.
# Generate system prompt for the summary agent
summary_prompt = """
You are an expert reasearcher in behaviour science and are tasked with summarising a reasearch panel. Please provide a structured summary of the key findings, including pain points, preferences, and suggestions for improvement.
This should be in the format based on the following format:```
Reasearch Study: <<Title>>
Subjects:
<<Overview of the subjects and number, any other key information>>
Summary:
<<Summary of the study, include detailed analysis as an export>>
Pain Points:
- <<List of Pain Points - Be as clear and prescriptive as required. I expect detailed response that can be used by the brand directly to make changes. Give a short paragraph per pain point.>>
Suggestions/Actions:
- <<List of Adctions - Be as clear and prescriptive as required. I expect detailed response that can be used by the brand directly to make changes. Give a short paragraph per reccomendation.>>
```
"""
Definire l'agente di riepilogo e il relativo ambiente — Creiamo un mini ambiente per l'esecuzione dell'agente di riepilogo. Ciò richiederà il proprio proxy (ambiente) e il comando di avvio che estrarrà le trascrizioni (prompt_utente) come input.
summary_agent = autogen.AssistantAgent(
name="SummaryAgent",
llm_config=llm_config,
system_message=summary_prompt + generate_notice(),
)
summary_proxy = autogen.UserProxyAgent(
name="summary_proxy",
code_execution_config={"last_n_messages": 2, "work_dir": "groupchat"},
system_message="A human admin.",
human_input_mode="TERMINATE"
)
summary_proxy.initiate_chat(
summary_agent,
message=user_prompt,
)
Questo ci fornisce un output sotto forma di pagella in Markdown, insieme alla possibilità di porre ulteriori domande in un chatbot in stile domande e risposte in cima ai risultati.
Fonte: towardsdatascience.com